Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 웹크롤러
- text
- texttheme
- map
- 파이썬
- crawler
- Collection
- 콜렉션
- List
- function
- Android
- 크롤러
- animation
- package
- ML
- 함수
- textstyle
- 클래스
- set
- import
- pushnamed
- kotlin
- variable
- Class
- 플러터
- 코틀린
- Flutter
- DART
- python
- 다트
Archives
- Today
- Total
조용한 담장
코틀린(Kotlin) Collections : Aggregate Operations 본문
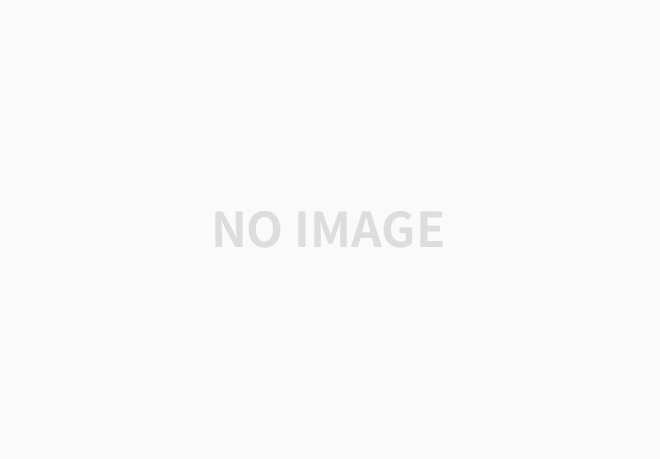
코틀린의 collection 의 Aggregate Operations 에 대해 살펴보자.
원문 https://kotlinlang.org/docs/reference/collection-aggregate.html을 보며 정리.
- min() and max() return the smallest and the largest element respectively;
- average() returns the average value of elements in the collection of numbers;
- sum() returns the sum of elements in the collection of numbers;
- count() returns the number of elements in a collection;
val numbers = listOf(6, 42, 10, 4)
println("Count: ${numbers.count()}")
println("Max: ${numbers.max()}")
println("Min: ${numbers.min()}")
println("Average: ${numbers.average()}")
println("Sum: ${numbers.sum()}")
// output:
// Count: 4
// Max: 42
// Min: 4
// Average: 15.5
// Sum: 62
- maxBy()/minBy() take a selector function and return the element for which it returns the largest or the smallest value.
- maxWith()/minWith() take a Comparator object and return the largest or smallest element according to that Comparator.
val numbers = listOf(5, 42, 10, 4)
val min3Remainder = numbers.minBy { it % 3 }
println(min3Remainder)
val strings = listOf("one", "two", "three", "four")
val longestString = strings.maxWith(compareBy { it.length })
println(longestString)
// output:
// 42
// three
- sumBy() applies functions that return `Int` values on collection elements.
- sumByDouble() works with functions that return `Double`.
val numbers = listOf(5, 42, 10, 4)
println(numbers.sumBy { it * 2 })
println(numbers.sumByDouble { it.toDouble() / 2 })
// output:
// 122
// 30.5
Fold and reduce
reduce() 와 fold() 는 주어진 동작을 collection 의 모든 element 에 연속적으로 적용한 결과깂들을 합친 결과물을 얻을수 있다.
연속적으로 붙어있는 두개의 element (앞의 element 까지 합쳐진 결과물과 현재의 새로운 대상) 을 argument 로 받아 연속적으로 동작을 수행한다.
두 함수의 차이는 fold() 는 initial value 를 받아 첫 element 와 함께 동작을 시작하고 reduce() 는 element 의 첫번째, 두번째 element 를 가지고 동작을 시작한다.
val numbers = listOf(5, 2, 10, 4)
val sum = numbers.reduce { sum, element -> sum + element }
println(sum)
val sumDoubled = numbers.fold(1) { sum, element -> sum + element }
println(sumDoubled)
// output:
// 21
// 22
reduceRight() 와 foldRight() 를 사용하여 마지막 element 부터 시작하는 반대 순서로 동작을 수행한다.
val sum = numbers.reduceRight() { sum, element -> sum + element }
val sumDoubled = numbers.foldRight(1) { sum, element -> sum + element }
reduceIndexed() 와 foldIndexed() 를 사용하면 element 의 인덱스 정보를 사용한 동작을 수행할 수 있다.
reduceRightIndexed() 와 foldRightIndexed() 를 사용하여 반대의 순서로 수행할 수 있다.
val numbers = listOf(5, 2, 10, 4)
val sumEven = numbers.foldIndexed(0) { idx, sum, element -> if (idx % 2 == 0) sum + element else sum }
println(sumEven)
val sumEvenRight = numbers.foldRightIndexed(0) { idx, element, sum -> if (idx % 2 == 0) sum + element else sum }
println(sumEvenRight)
// output:
// 15
// 15
'kotlin' 카테고리의 다른 글
코틀린(Kotlin) Collections : List Specific Operations (0) | 2020.05.07 |
---|---|
코틀린(Kotlin) Collections : Write Operations (0) | 2020.04.14 |
코틀린(Kotlin) Collections : Ordering (0) | 2020.04.07 |
코틀린(Kotlin) Collections : Retrieving Single Elements (0) | 2020.04.06 |
코틀린(Kotlin) Collections : Retrieving Collection Parts (0) | 2020.04.06 |